Bubble Sort is the simple sorting algorithm for array that works by repeatedly swapping the adjacent elements if they are in the wrong order i.e Low to High.
This algorithm is not preferable for large data sets as its worst-case time complexity is very high.
Its an algorithm that sorts the array by comparing two adjacent elements and swaps them if they are not in the intended order
Lets take an examples so we can understand its working
We have an unsorted array arrayData = [778, 78, 789, 3, 99, 2786, 0, 499] the task is to sort the array using bubble sort.
Bubble sort compares the element from index 0 and if the 0th index is greater than 1st index then the values get swapped and
if the 0th index is less than the 1st index then nothing will change.
After that, the 1st index compares to the 2nd index then the 2nd index compares to the 3rd, and process going on till the last index.
Syntax:
bubbleSort(array) {
for i -> 0 to arrayLength
for j -> 0 to (arrayLength - i - 1)
if arrayData[j] > arrayData[j + 1]
swap(arrayData[j], arrayData[j + 1])
}
Bubble sort Implementation using Javascript
//# Creating the bblSort function
function bblSort(arrayData){
for(var i = 0; i < arrayData.length; i++){
//# Last i elements are already in place
for(var j = 0; j < ( arrayData.length - i -1 ); j++){
//# Checking if the item at present iteration
//# is greater than the next iteration
if(arrayData[j] > arrayData[j+1]){
//# If the condition is true then swap them
var temp = arrayData[j]
arrayData[j] = arrayData[j + 1]
arrayData[j+1] = temp
}
}
}
//# Print the sorted array
console.log(arrayData);
}
//# This is our unsorted array
var arrayData = [778, 78, 789, 3, 99, 2786, 0, 499];
//# Now pass this array to the bblSort() function
bblSort(arrayData);
Execution on developer Console
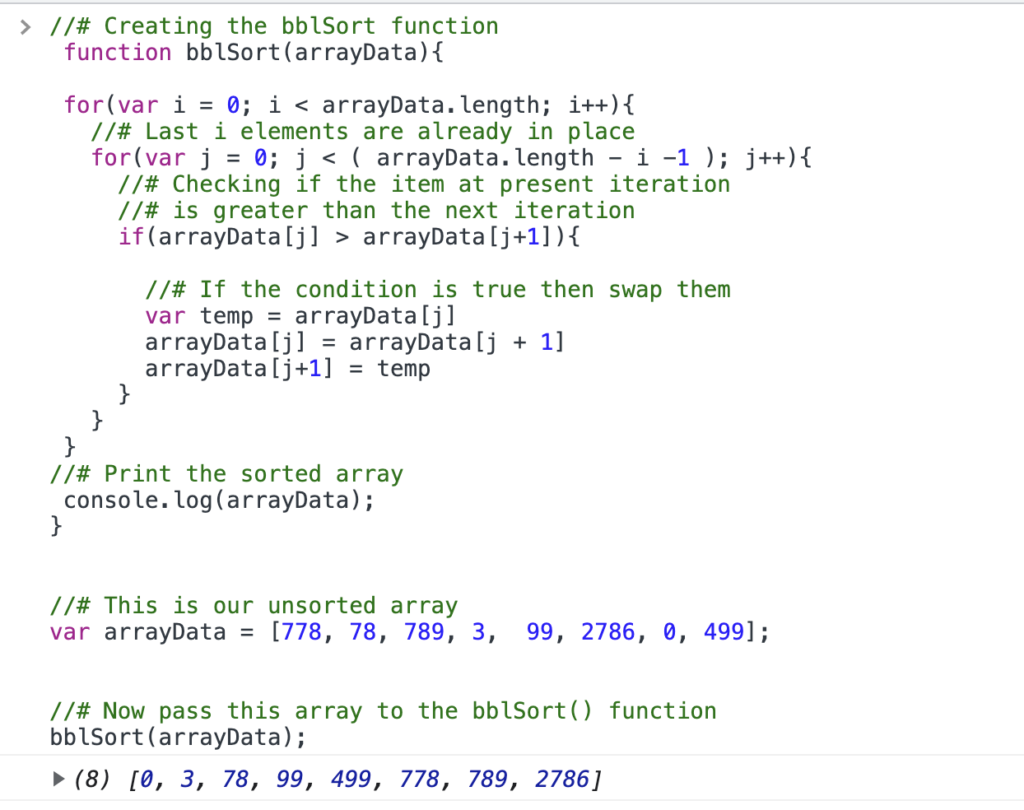