Git Commands and Shortcuts
GitHub is a code hosting platform for version control and collaboration.
It lets you and others work together on projects from anywhere.
This commands teaches you GitHub essentials commands for repositories, branches, commits, and pull requests.
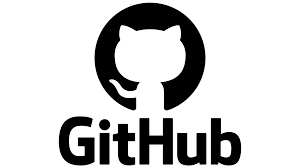
1. git init
For re-initialise branch after copy paste repo in system, you can reinitialise it
2. git config –global alias.mylog ‘!git log –author=”$(git config user.name)”‘
show only my-log by git my-log
You can use command
3. git log –author=”<Your-Username>”
git log –author=”” To Check log by author
4. git status
Show modified files in working directory, staged for your next commit
5. git add [file]
add a file as it looks now to your next commit (stage)
6. git reset [file]
Unstage a file while retaining the changes in working directory
7. git reset –hard
Discard all local changes to all files permanently
8. git show <commitID/b626a2255b658f7de69c92cd58bc8eb1226353f9>
show all commit code of comited hash
9. git show –pretty=”” –name-only b626a2255b658f7de69c92cd58bc8eb1226353f9
show all commit code of committed hash
10. git cherry
To check all local commit hash-code
11.git log
To check all logs
12. git revert
For revert commit
13. git config –global credential.helper ‘cache –timeout 14400’
14. git clone
For take clone of git repo through URL
15. git –version
For check version
16. git checkout
for revert un-staged or non added files
17. git reset
for revert staged to un-staged file
18. git log -p app.module.core.js
To check log of a file
19. git log app.module.core.js
To check log of a file
20. git stash
For stash all modified local changes
21. git stash pop
For revert stash files and code
22. git log –committer=”<committer-name>”
To check log by committer
23. git config –list
To check all global config
24. git config –global user.name “<user-name>”
It is used to set user.name
25. git config –global user.email “<user-email>”
It is used to set user.email
26. git log –diff-filter=D –summary
It is used to get all deleted history
27. git log –diff-filter=D –summary | grep -A 15 abcd
It is use to get all deleted history and find text abcd
28. git log –diff-filter=D –summary | grep abcd
It is use to get all deleted history and find text abcd
29. git log –all –full-history
It is use to get all history
30. git log –all –full-history
It is use to get history of files
31. ls -al
list hidden files
32. git push –set-upstream origin feature_dev_backup
It is use to push new branch to github
33. git branch
It is list your branches and* will appear next to the currently active branch
34. git branch [branch-name]
create a new branch at the current commit
35. git checkout
Switch to another branch and check it out into your working directory
36. git merge [branch]
Merge the specified branch’s history into the current one
37. git log –oneline
for shorter version
38. git clean -df
For clean and remove all un-staged files
39. git rebase –abort
For * (no branch, rebasing feature branch) issue
40. git cherry-pick
For specific changes commit of feature branch to dev commit
git cherry-pick hashcode of feature branch commit
git checkout dev
git pull
git push for commit via cherrypick
41. git –help
For git command helps
42. Delete a remote branch
$ git push origin –delete
# Git version 1.7.0 or newer
$ git push origin
# Git versions older than 1.7.0
43. Delete a local branch
$ git branch –delete
$ git branch -d
# Shorter version
$ git branch -D
# Force delete un-merged branches
44. Delete a local remote-tracking branch
$ git branch –delete –remotes /
$ git branch -dr /
# Shorter
45. $ git fetch –prune
# Delete multiple obsolete tracking branches
46. $ git fetch -p
# Shorter” delete branch
47. Git merge commands
git checkout -b branchName $(git merge-base dev staging)
git checkout dev
git merge branchName
git checkout staging
git merge branchName
git checkout -b branchName $(git merge-base dev staging) //for creating branch
//work on branch and push to feature
git checkout dev //move dev
git pull –rebase
git merge branchName //merge branchName to dev
git checkout staging //move to staging
git pull –rebase
git merge branchName //merge branchName to staging” for merge
For Windows. Run these commands in Git Bash:
git config –global diff.tool meld
git config –global difftool.meld.path “C:\Program Files (x86)\Meld\Meld.exe”
git config –global difftool.prompt false
git config –global merge.tool meld
git config –global mergetool.meld.path “C:\Program Files (x86)\Meld\Meld.exe”
git config –global mergetool.prompt false
(Update the file path for Meld.exe if yours is different.)
For Linux. Run these commands in Git Bash:
git config –global diff.tool meld
git config –global difftool.meld.path “/usr/bin/meld”
git config –global difftool.prompt false
git config –global merge.tool meld
git config –global mergetool.meld.path “/usr/bin/meld”
git config –global mergetool.prompt false
You can verify Meld’s path using this command:
which meld” for set meld and diff tool
alias skipconflict=” git reset –hard ;
git clean -fd;
git rebase –skip ” for skipconflict
git checkout mybranch
48. git reset –hard origin/mybranch”
for reset branch
49. Deleting and creating new branch as script
“echo “switch to dev branch for perform operation”;
git checkout dev;
git pull origin dev;
echo “performing operation…”
echo “deleting feature_merge_dev_staging branch…”;
git push origin –delete feature_merge_dev_staging;
git branch –delete feature_merge_dev_staging;
echo “feature_merge_dev_staging branch deleted.”;
echo “deleting feature_merge_dev…”;
git push origin –delete feature_merge_dev;
git branch -D feature_merge_dev;
echo “feature_merge_dev branch deleted.”;
echo “deleting feature_merge_staging…”;
git push origin –delete feature_merge_staging;
git branch -D feature_merge_staging;
echo “feature_merge_staging branch deleted.”;
echo “creating feature_merge_dev branch…”;
git checkout dev;
git pull origin dev;
git checkout -b feature_merge_dev;
git push –set-upstream origin feature_merge_dev;
echo “feature_merge_dev branch created.”;
echo “creating feature_merge_staging branch…”;
git checkout staging;
git pull origin staging;
git checkout -b feature_merge_staging;
git push –set-upstream origin feature_merge_staging;
echo “feature_merge_staging branch created.”;
echo “creating feature_merge_dev_staging branch…”;
git checkout -b feature_merge_dev_staging $(git merge-base dev staging);
git push –set-upstream origin feature_merge_dev_staging;
echo “feature_merge_dev_staging branch created.”;
Above script to recreate feature branches
50. git checkout -b feature_merge_devstagmast $(git merge-base dev staging master);
Above script for code if you want commit on all branch
51. git clean -d -i
It is use to clean ” ” untracked files remove
52. git mergetool
Used for for merge purpose
53. git –version
Use to check git version
54.”sudo add-apt-repository -y ppa:git-core/ppa
sudo apt-get update
sudo apt-get install git -y”
Update git version
55. git remote -v
Check URL of git
56. git remote -v
origin http://103.204.52.2:33880/ui/app-ui (fetch)
origin http://103.204.52.2:33880/ui/app-new-ui (push)
57. git clone –single-branch –branch
ex: git clone –single-branch –branch staging http://172.16.1.140:88/app/ui.git
For Single branch checkout
58. git fetch
It is use to fetch all committed pushed git branches
59. git reset –hard HEAD~1
It is use to revert local commit
60. git config –global pull.rebase true
It is use for rebase globally in checkout
61. git reset –hard origin/dev
It is use to reset local commit
62. git pull –rebase OR git pull
To take pull of branch
63. git commit -m “feat: commit code”
To commit the code